Understanding Basic Algorithms: A Friendly Guide for Beginners
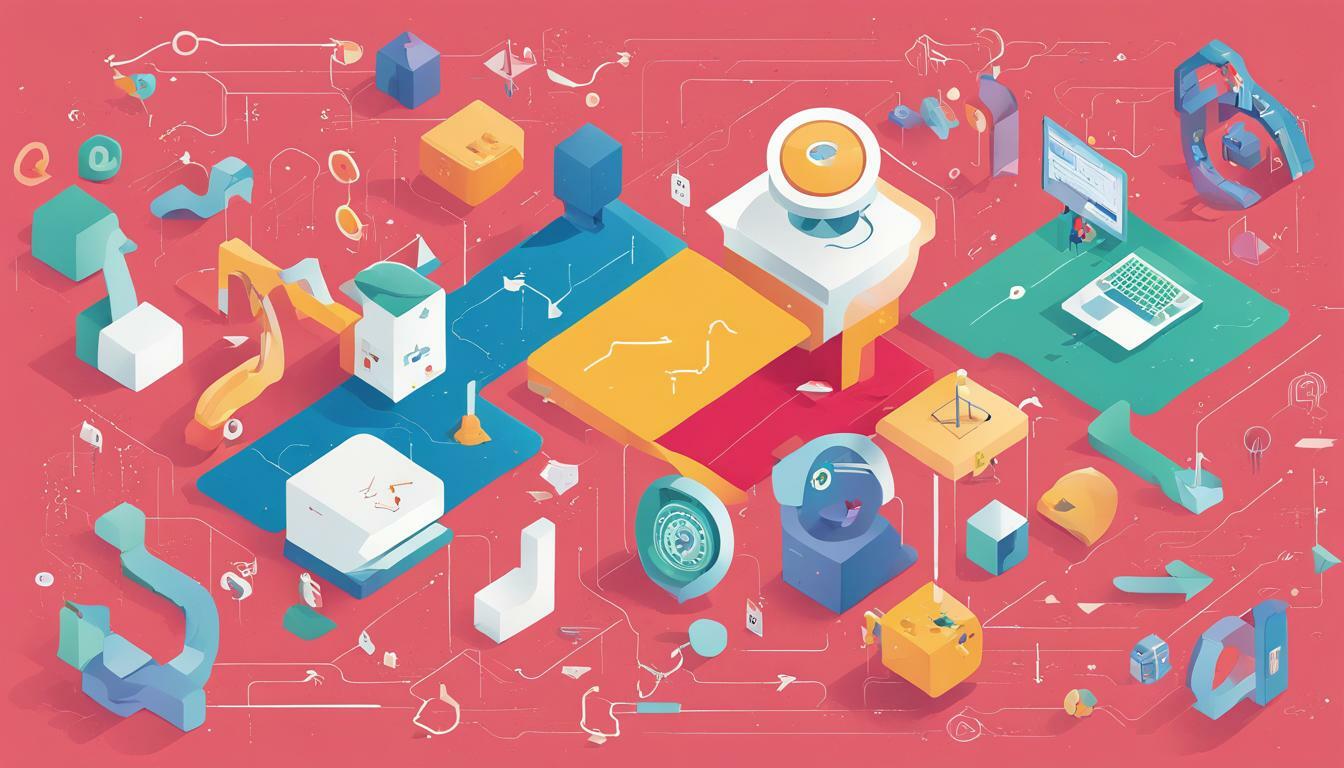
Having a solid background in journalism and copywriting, I appreciate the hurdles that newcomers encounter while attempting to grasp algorithms. The labyrinth of intricate terminology and complicated theories indeed poses a formidable barrier, evoking unease. Acknowledging this, I’ve developed a guide specifically designed for beginners to simplify algorithms in the most straightforward way imaginable.
Whether you’re a student or a professional in any field, understanding algorithms is essential in today’s data-driven world. Algorithms are used everywhere, from social media and e-commerce to healthcare and finance. They are the foundation of computing and enable us to solve complex problems efficiently.
In this section, we’ll cover the basics of algorithms and help you get started on your journey to becoming proficient in algorithmic problem-solving.
Key Takeaways
- Algorithms are essential in today’s data-driven world and are used in various domains.
- Basic algorithms enable us to solve complex problems efficiently.
- Understanding algorithms is crucial for students and professionals in any field.
What Are Algorithms?
In the world of computer science, algorithms are essential. They are the basis on which we build software systems and solve complex problems. In simple terms, an algorithm is a set of steps or instructions to perform a specific task. These tasks can range from solving mathematical problems to searching for data in databases.
At its core, an algorithm is a logical sequence of steps that must be completed to solve a problem. It takes an input, performs a set of operations, and produces an output. The input can be anything from data to a problem to be solved, while the output is a solution or a result of the algorithm’s operations.
Algorithms are everywhere, from the apps we use on our phones to the software we rely on for work. They are used to sort information, search for data, and even create complex animations. Understanding algorithm concepts and algorithm fundamentals is essential to becoming proficient in computer science.
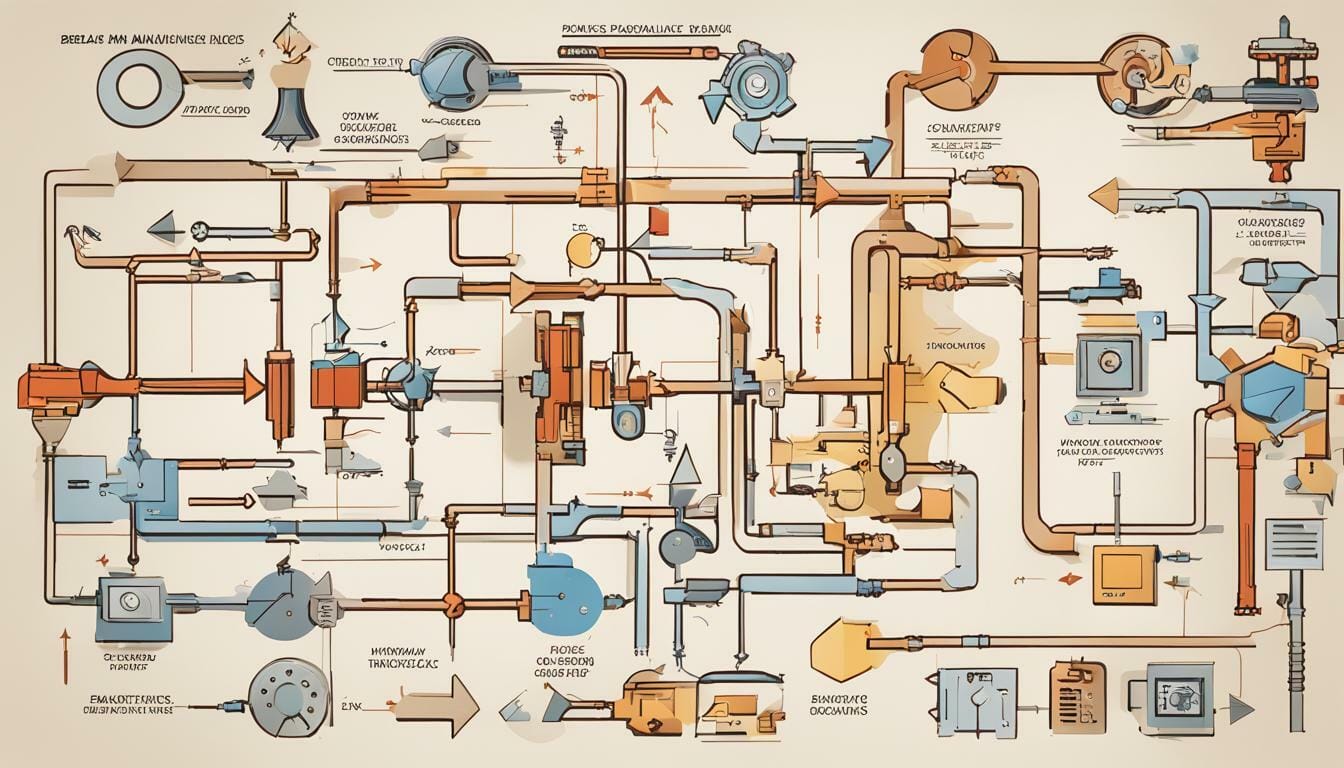
Some essential algorithms that form the foundation of computer science include:
- Sorting Algorithms: These algorithms arrange data in a specific order, such as numerical or alphabetical. Examples include bubble sort and quicksort.
- Searching Algorithms: These algorithms find specific data in a dataset. Examples include binary search and linear search.
- Pathfinding Algorithms: These algorithms are used to find the shortest path between two points. Examples include Dijkstra’s algorithm and A* algorithm.
- Encryption Algorithms: These algorithms convert plaintext into ciphertext, making it unreadable to anyone without the proper decryption key. Examples include RSA and AES.
By understanding algorithm concepts and essential algorithms, beginners can gain a solid foundation in computer science and algorithmic problem-solving.
The Basics of Algorithm Design
As a beginner, understanding basic algorithmic principles is essential. Algorithm design involves breaking down a complex problem into smaller, manageable subproblems. The subproblems are then solved systematically, leading to the solution of the original problem.
There are three key components of algorithm design: input, output, and control structures. The input is the data that the algorithm receives and processes. The output is the result of the algorithm’s processing. Control structures determine how the algorithm processes the input and generates the output.
When designing algorithms, it’s important to consider their efficiency. An algorithm’s efficiency refers to the resources it requires to run, such as time and memory. Efficient algorithms are those that require fewer resources and can handle large amounts of data inputs.
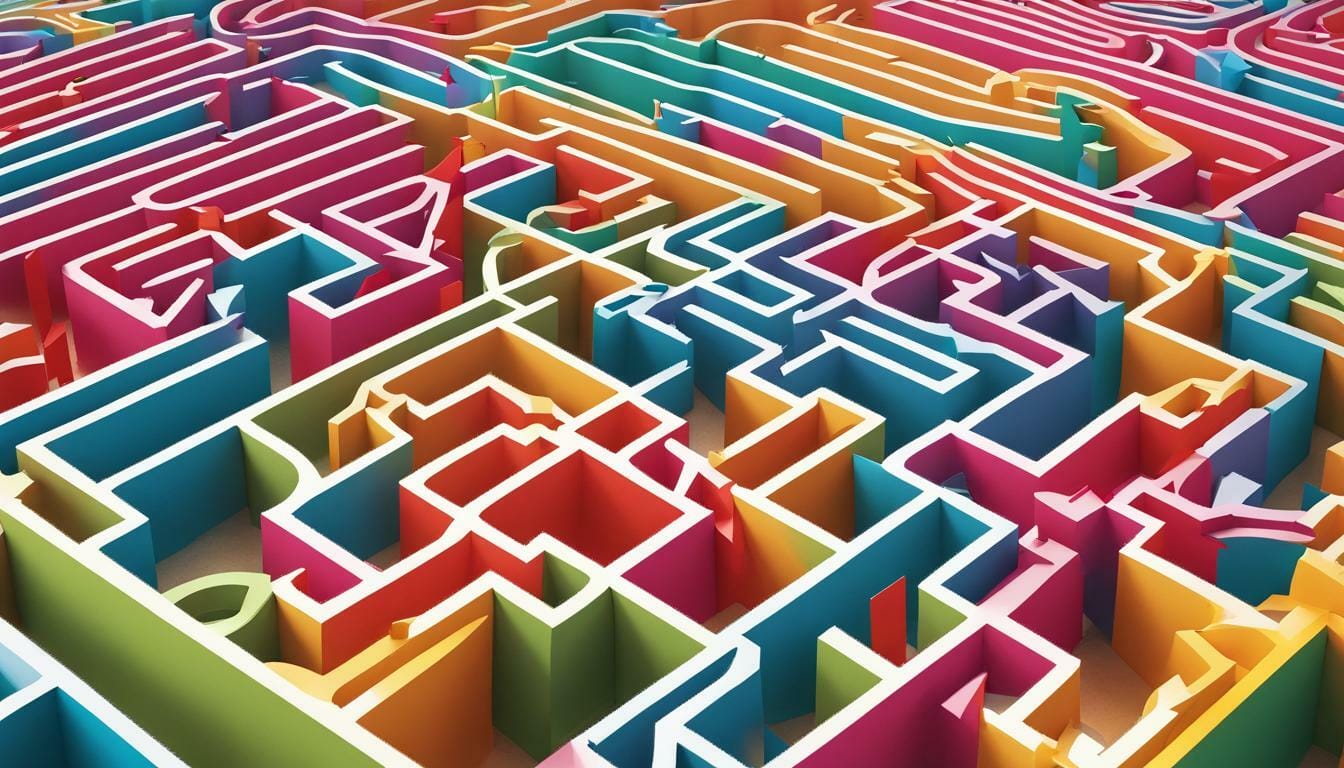
There are several algorithm basics for beginners to keep in mind when designing algorithms. First, algorithms should be simple and easy to follow. Complexity should be avoided whenever possible. Second, algorithms should be scalable so that they can handle larger input sizes. Third, algorithms should be modular so they can be reused across different programs or projects. Finally, algorithms should be easy to test and debug to ensure they work as intended.
Understanding Algorithm Complexity
One of the most crucial aspects of algorithm design is understanding the concept of algorithm complexity. The efficiency of an algorithm depends on its input size, and analyzing this relationship is integral to selecting the most appropriate algorithm for a given problem.
There are two main types of algorithmic complexity: time complexity and space complexity. Time complexity refers to the amount of time it takes an algorithm to run as a function of its input size. Space complexity, on the other hand, refers to the amount of memory an algorithm requires as a function of its input size.
Understanding algorithmic complexity is especially important when dealing with large datasets and complex problems. When designing algorithms for these scenarios, it’s essential to consider the tradeoff between time and space complexity, as optimizing one often comes at the expense of the other.
There are several techniques for analyzing and optimizing algorithm complexity. One common technique is to use “Big O” notation, which describes the upper bound of an algorithm’s time or space complexity in terms of its input size. For example, an algorithm with a time complexity of O(n) means that its runtime increases linearly with input size.
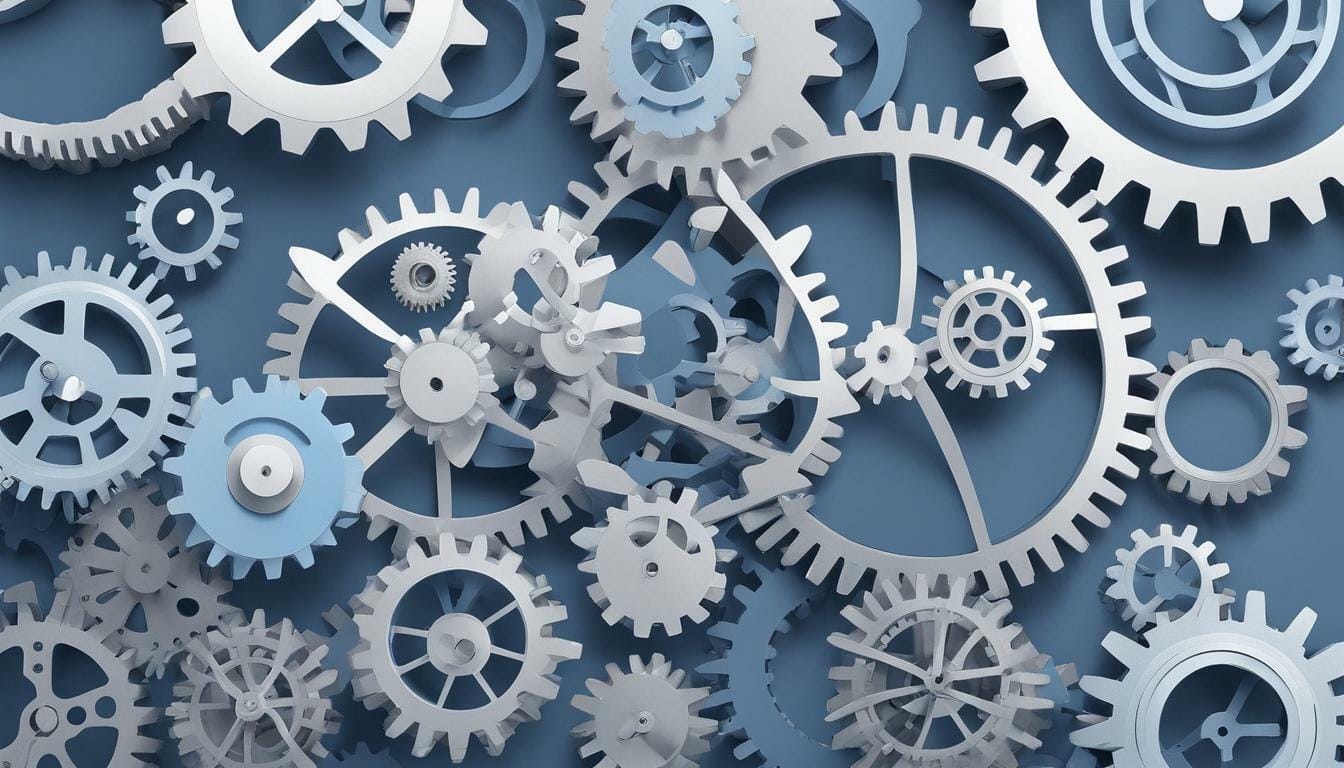
Another important concept in algorithm complexity is the notion of “fundamental algorithms.” Fundamental algorithms are a set of basic algorithms that are commonly used as building blocks for more complex algorithms. By understanding these fundamental algorithms, beginners can gain a solid foundation in algorithmic problem-solving.
Examples of fundamental algorithms include sorting algorithms like bubble sort and quicksort, and searching algorithms like linear search and binary search. By learning these algorithms and their time and space complexities, beginners can begin to develop a sense of how to select the most appropriate algorithm for a given problem.
Sorting and Searching Algorithms
Sorting and searching algorithms are fundamental to basic algorithms. They are the building blocks of many computer programs and are used in a variety of applications. In this section, we’ll explore some of the most important sorting and searching algorithms.
Bubble Sort
Bubble sort is one of the simplest sorting algorithms. It works by repeatedly swapping adjacent elements if they are in the wrong order. The algorithm takes its name from the way smaller elements “bubble” to the top of the list as the algorithm progresses.
The time complexity for bubble sort is O(n^2), which makes it inefficient for large datasets. However, it remains a useful algorithm for teaching sorting concepts and is a good choice for small datasets with minimal overhead.
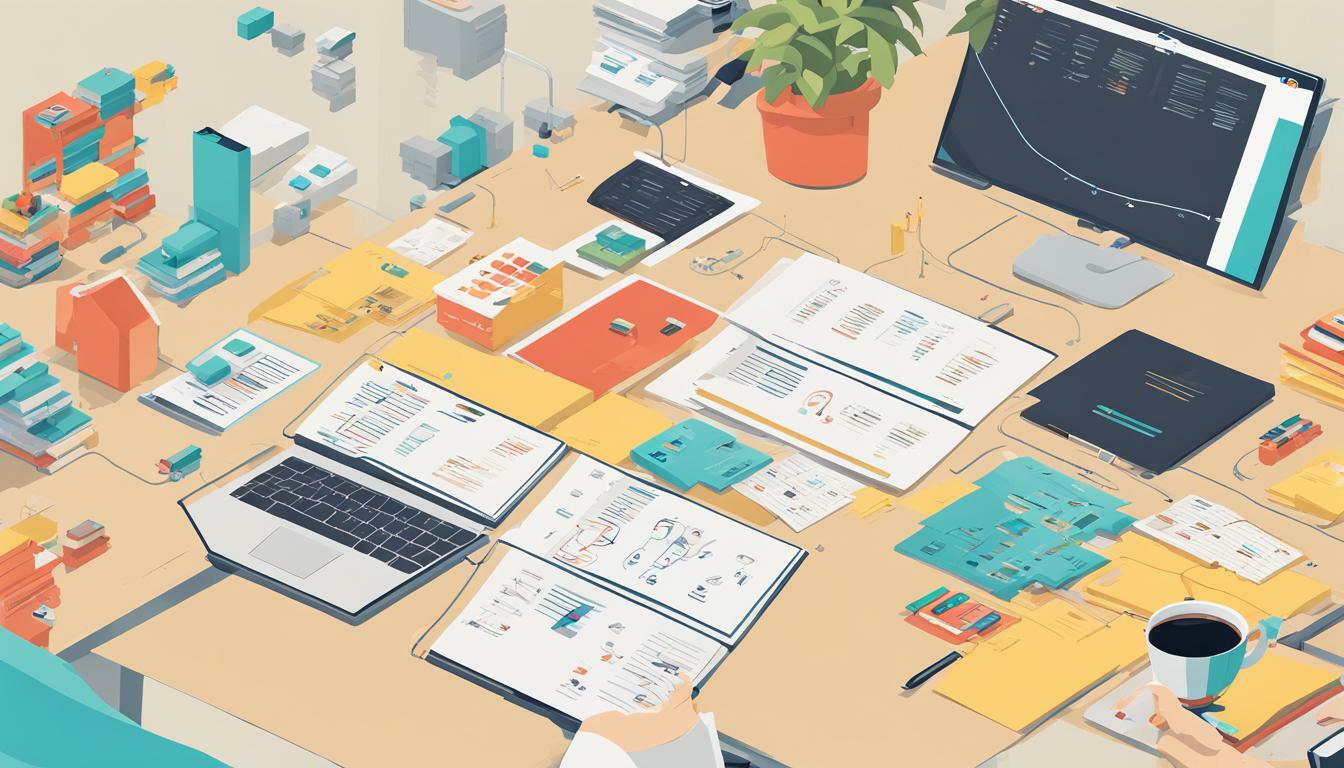
Insertion Sort
Insertion sort is another simple sorting algorithm. It works by iteratively placing each element in the correct location relative to its sorted position within the list. The algorithm is well suited for small datasets and has a time complexity of O(n^2). However, it becomes inefficient for larger datasets.
Binary Search
Binary search is a fundamental searching algorithm. It works by dividing the dataset in half at each step, then comparing the search value to the middle element of the current partition. If the search value is less than the middle element, the algorithm continues to search the left partition; otherwise, it searches the right partition. The algorithm repeats until the search value is found or the dataset is exhausted.
Binary search has a time complexity of O(log n), making it efficient for large datasets. It is commonly used in applications such as databases and search engines.
Linear Search
Linear search is a simple searching algorithm that works by iterating through the dataset one element at a time, comparing each element to the search value. The algorithm continues until the search value is found or the dataset is exhausted.
Linear search has a time complexity of O(n), which makes it inefficient for large datasets. However, it remains a useful algorithm for small datasets and has applications in fields such as data validation and error checking.
Recursion and Iteration in Algorithms
Understanding the concepts of recursion and iteration is crucial in solving complex problems using algorithms. Recursion is a process in which a function or routine calls itself repeatedly until it reaches a base case. In contrast, iteration is a process in which a loop is used to repeat a set of instructions until a specified condition is met.
Recursion and iteration have their own advantages and disadvantages, and choosing one over the other depends on the specific problem being solved. Recursion is often more elegant and intuitive, but it can consume more memory as each recursive call creates a new instance of the function. Iteration, on the other hand, is often more efficient in terms of memory usage but may be less intuitive as it requires manual management of the loop.
One common use of recursion in algorithms is in sorting and searching. For example, the quicksort algorithm uses recursion to divide a large array into smaller subarrays, sort them recursively, and then merge them back together. In contrast, the binary search algorithm uses iteration to repeatedly narrow down the search space until the desired item is found.
It’s essential to understand both recursion and iteration in order to be a proficient algorithm designer and problem solver. Being able to choose the appropriate method for solving a problem can make the difference between an efficient and inefficient algorithm.
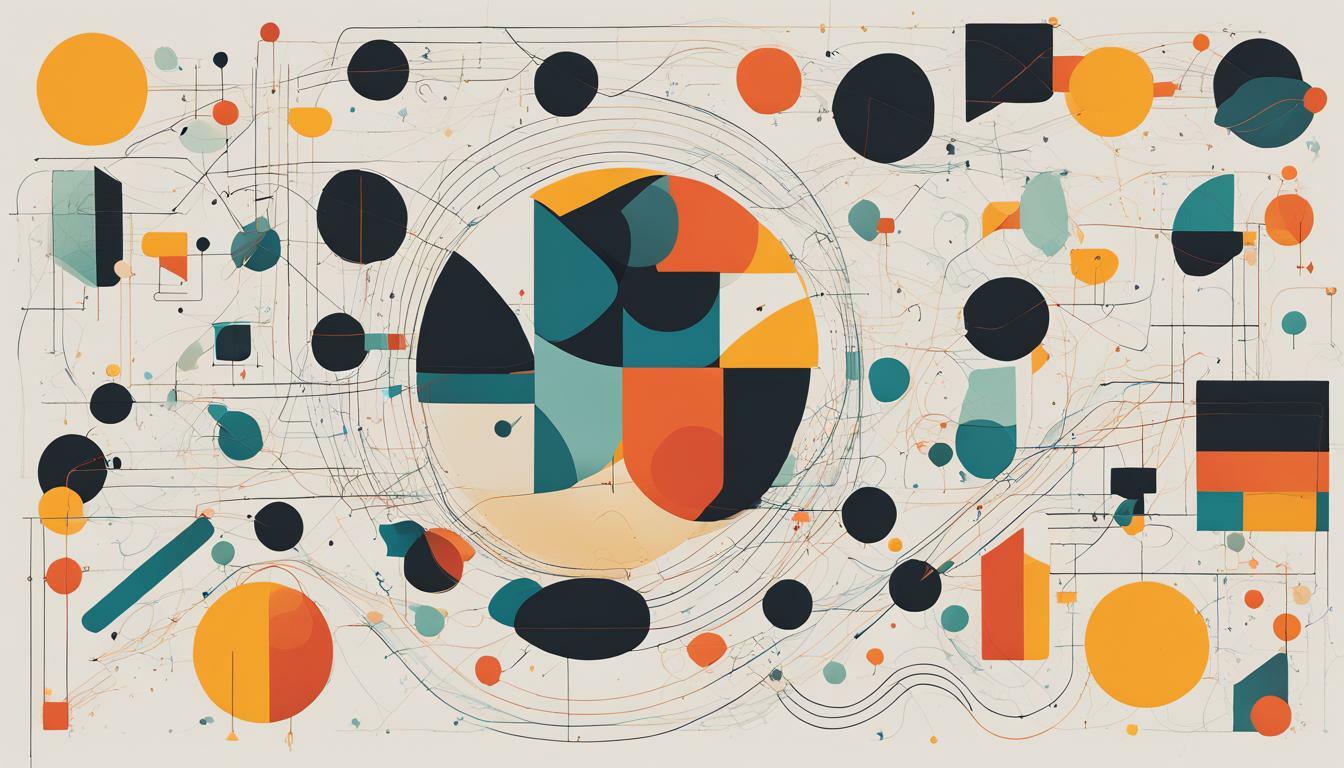
Popular Algorithms and their Applications
As we’ve seen, there are numerous essential algorithms in computer science. Many of these algorithms have real-world applications in various industries. Let’s take a look at some of the most popular algorithms and their applications.
Dijkstra’s Algorithm
Essential algorithms, algorithm fundamentals
Dijkstra’s algorithm is a pathfinding algorithm that is used to find the shortest path between two nodes on a graph. It has many applications in transportation routing, network routing, and other fields. For example, it is commonly used by GPS systems to find the shortest route between two locations.
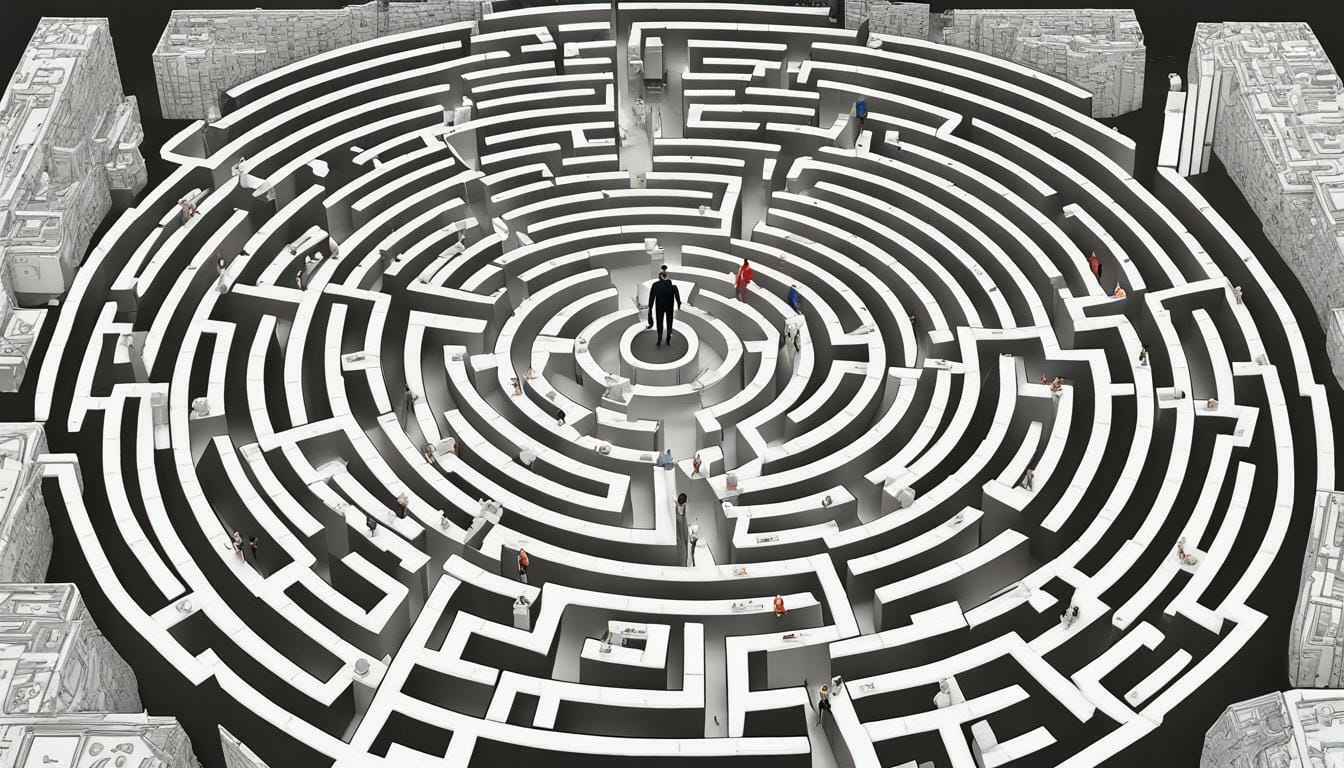
Breadth-first Search
Essential algorithms, algorithm fundamentals
Breadth-first search is a graph traversal algorithm that explores all the vertices of a graph in breadth-first order. It has many applications in network analysis, social network analysis, and web crawling. For example, it is used by search engines like Google to crawl and index web pages.
Quicksort
Essential algorithms, algorithm fundamentals
Quicksort is a sorting algorithm that is widely used in computer science. It has many applications in databases, operating systems, and other fields where sorting large amounts of data is required. For example, it is used to sort search results on e-commerce websites.
By understanding these popular algorithms and their applications, beginners can gain a deeper appreciation for the importance of algorithms in computer science and beyond.
Is Knowledge of Basic Algorithms Necessary to Learn Full Stack Development?
To grasp the intricacies of full stack development, acquiring knowledge of basic algorithms is crucial. Understanding algorithms facilitates efficient problem-solving, enabling developers to create optimized solutions. While the learn full stack development duration may vary for each individual, a strong foundation in algorithms significantly enhances the learning process and equips developers with the necessary skills to tackle complex projects effectively.
Algorithm Efficiency and Optimization Techniques
Algorithm efficiency is a key factor in problem-solving, especially for large or complex datasets. As a beginner, there are several optimization techniques you can use to enhance the efficiency of your algorithms.
One of the fundamental concepts of algorithm efficiency is algorithmic complexity. This is a measure of the computational resources required by an algorithm, usually with respect to input size. For instance, an algorithm that requires more computation time or memory for larger datasets has a higher complexity.
Understanding algorithmic complexity is essential for selecting the best algorithm for a particular task. As a beginner, you can use basic algorithms with simple and easy-to-understand operations such as sorting and searching algorithms. However, as the dataset size increases, you may need more complex algorithms that can handle large amounts of data without degrading performance.
Another optimization technique is the space-time tradeoff. This technique involves optimizing the use of memory and computation time. In some cases, you can reduce the computation time by using more memory, or vice versa. For example, using a hash table or a lookup table can reduce the computation time of certain algorithms while increasing their memory usage.
Algorithmic optimization is also an important technique to enhance efficiency. This involves optimizing the algorithm’s source code to make it more efficient. For instance, you can optimize an algorithm by using a more suitable data structure or by restructuring the code to minimize redundant operations.
Overall, learning these optimization techniques is crucial for beginners in algorithmic problem-solving. By understanding algorithmic complexity, space-time tradeoff, and algorithmic optimization, you can enhance the efficiency and scalability of your algorithms, making them more effective in solving complex problems.
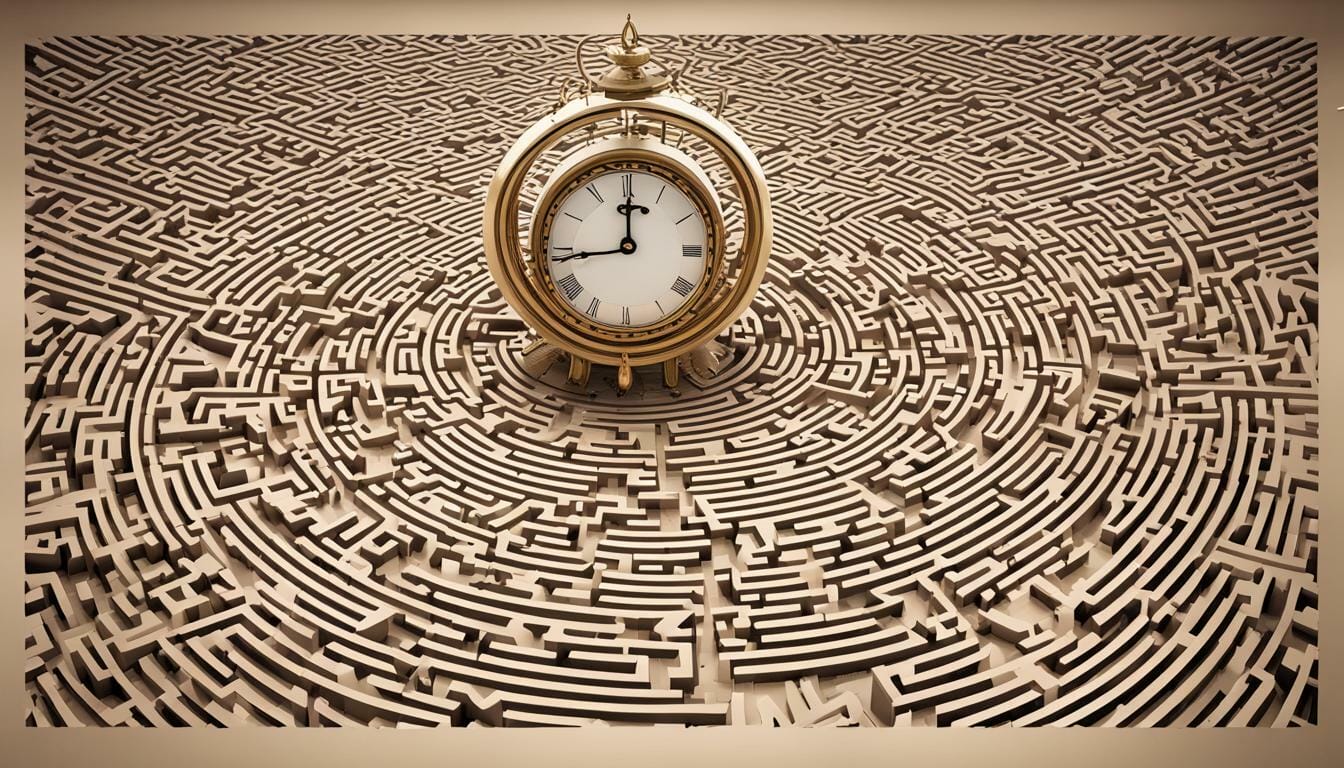
Conclusion
As we wrap up this beginner’s guide to basic algorithms, we hope that you now have a solid understanding of the fundamentals. We’ve covered the essential concepts and principles of algorithms, algorithm design, complexity analysis, and optimization techniques.
By grasping the basics, you are now equipped to tackle more complex algorithms and realize their practical applications. Algorithmic problem-solving skills are essential in computer science and many other fields, and mastering them can open up numerous opportunities.
Remember that the journey towards becoming proficient in algorithms is a lifelong pursuit. It takes time, patience, and practice. Continue to learn and explore new algorithms, and don’t be afraid to experiment with different approaches.
Thank you for taking the time to read this guide. We hope it has been a helpful resource in your journey towards understanding basic algorithms.
Keep exploring and have fun!
FAQ
Q: What are algorithms?
A: Algorithms are step-by-step instructions or procedures that solve problems. They provide a clear and systematic approach to problem-solving, breaking down complex tasks into smaller, more manageable parts.
Q: Why are algorithms important?
A: Algorithms are essential in computer science and programming. They are used to solve a wide range of problems, from sorting and searching data to performing complex mathematical calculations. Understanding algorithms is crucial for efficient and effective problem-solving.
Q: What are the basics of algorithm design?
A: The basics of algorithm design include determining the input and output of the algorithm, as well as choosing appropriate control structures. It’s essential to design algorithms that are efficient, scalable, and well-structured to solve problems effectively.
Q: How do you analyze algorithm complexity?
A: Algorithm complexity is analyzed by measuring how the algorithm’s performance changes with different input sizes. This analysis helps determine the algorithm’s efficiency and scalability. Common methods of analyzing algorithm complexity include time complexity and space complexity.
Q: What are some popular sorting and searching algorithms?
A: Some popular sorting algorithms include bubble sort, insertion sort, and quicksort. For searching, common algorithms are binary search and linear search. These algorithms are fundamental in organizing and retrieving data efficiently.
Q: What is the difference between recursion and iteration?
A: Recursion and iteration are both methods of repetition in algorithms. Recursion involves a function calling itself, while iteration uses loops to repeat a set of instructions. Both methods have their uses, and understanding when to use each is important for solving different types of problems.
Q: Can you provide examples of popular algorithms and their applications?
A: Sure! Dijkstra’s algorithm is used in finding the shortest path in a graph, breadth-first search helps in traversing or searching a graph, and quicksort is a widely-used sorting algorithm. These algorithms have numerous applications in various domains, such as network routing, data analysis, and more.
Q: How can algorithm efficiency be optimized?
A: There are various techniques to optimize algorithm efficiency. Examples include improving the algorithm’s time complexity, using data structures that suit the problem, or employing space-time tradeoffs. Optimization techniques help improve the performance of algorithms and enhance problem-solving capabilities.