Print Variables in javascript: Perfecting the Print to Console Function in 2024
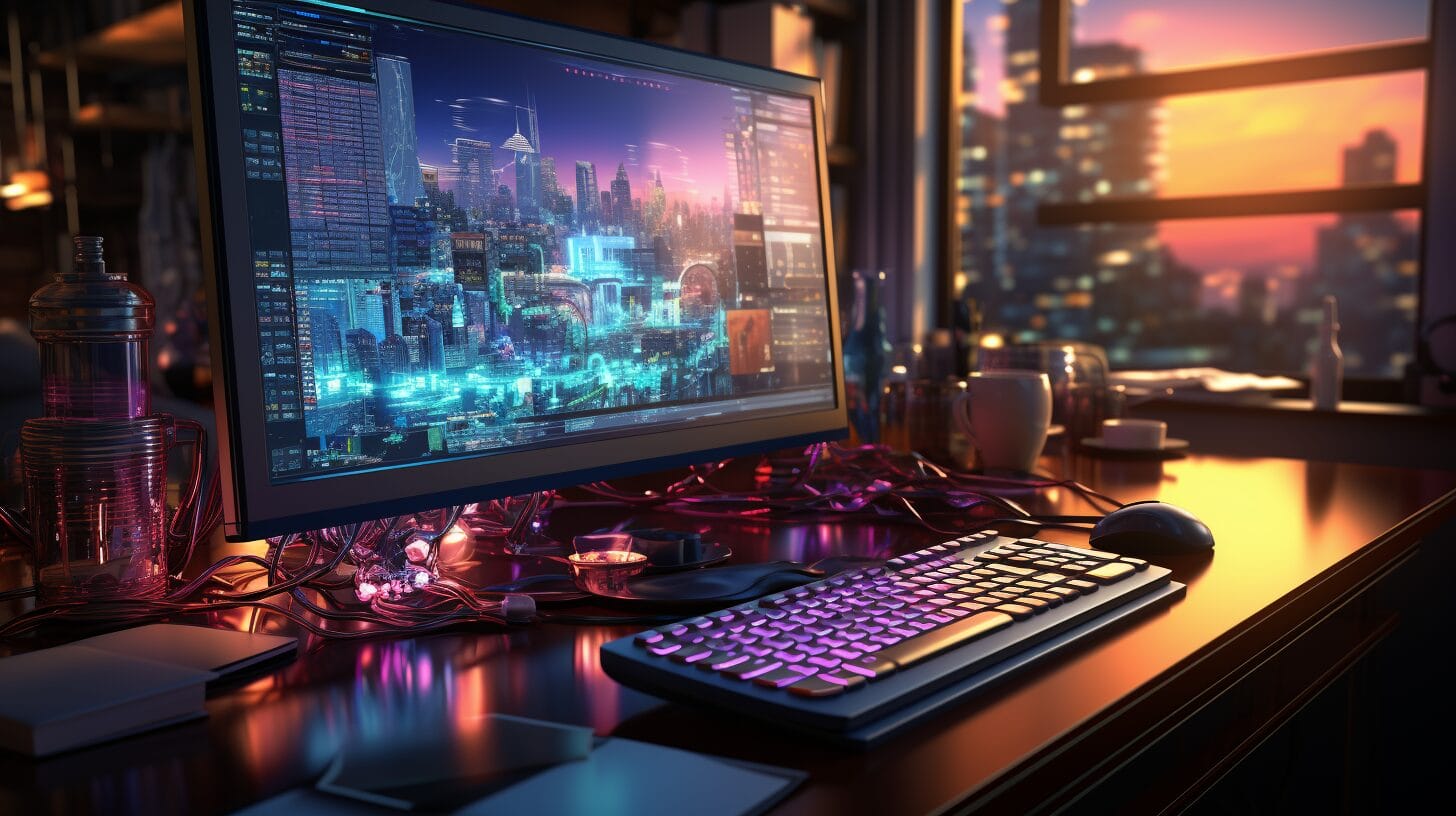
In the realm of web development, the console log function may not maintain the same appeal as other JavaScript elements, but mastering it is crucial. It offers a concealed route to manage complex coding challenges, acting as a subtle yet powerful ally.
Our exploration into the subtleties of print variables in JavaScript and the print-to-console function aims to deepen our understanding of the communication between code and the applications it powers. We will dissect best practices, unveil shortcuts, and reveal how to harness the full potential of console functions—not only for debugging but also for crafting eloquent code.
Join us as we refine our coding techniques and uncover the art of engaging with our code through the console.
Key Takeaways
- Variables in JavaScript dynamically store and manage data.
- The
var
,let
, andconst
keywords declare variables with distinct scopes and constraints. - Proper naming conventions for variables enhance code legibility and minimize errors.
- Arrays in JavaScript hold multiple values in one variable, enabling efficient data management.
Understanding Variables in JavaScript for Web Development
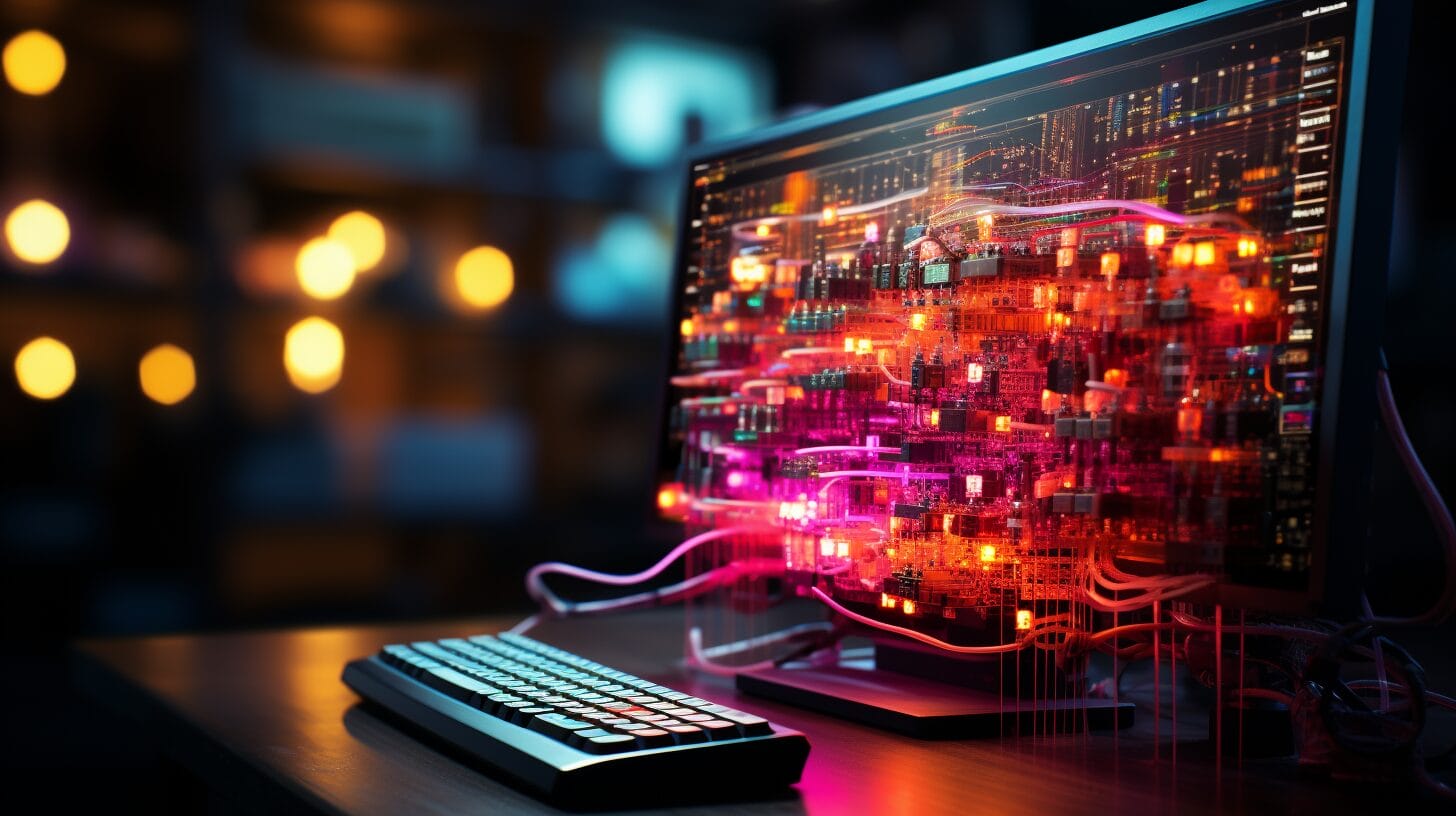
Variables in JavaScript are the bedrock for dynamically storing, updating, and managing data within code. Creating a variable is analogous to claiming a plot of digital real estate where we can construct our virtual edifices.
JavaScript offers three keywords for variable declaration—var
, let
, and const
—each providing different levels of scope and immutability. Var
is the traditional approach with function scope, while let
and const
offer block scope, const
ensuring the value of a variable remains unchanged.
Clarity in variable naming is crucial, akin to the careful selection of a child’s name, as it guides us to a clearer comprehension of the variable’s contents. This practice safeguards against confusion and errors.
Arrays in JavaScript serve as versatile containers capable of storing an array of values and streamlining data management tasks such as filtering, sorting, and iterating.
Interactive Tutorial on How to Declare and Assign Variable Values in JavaScript
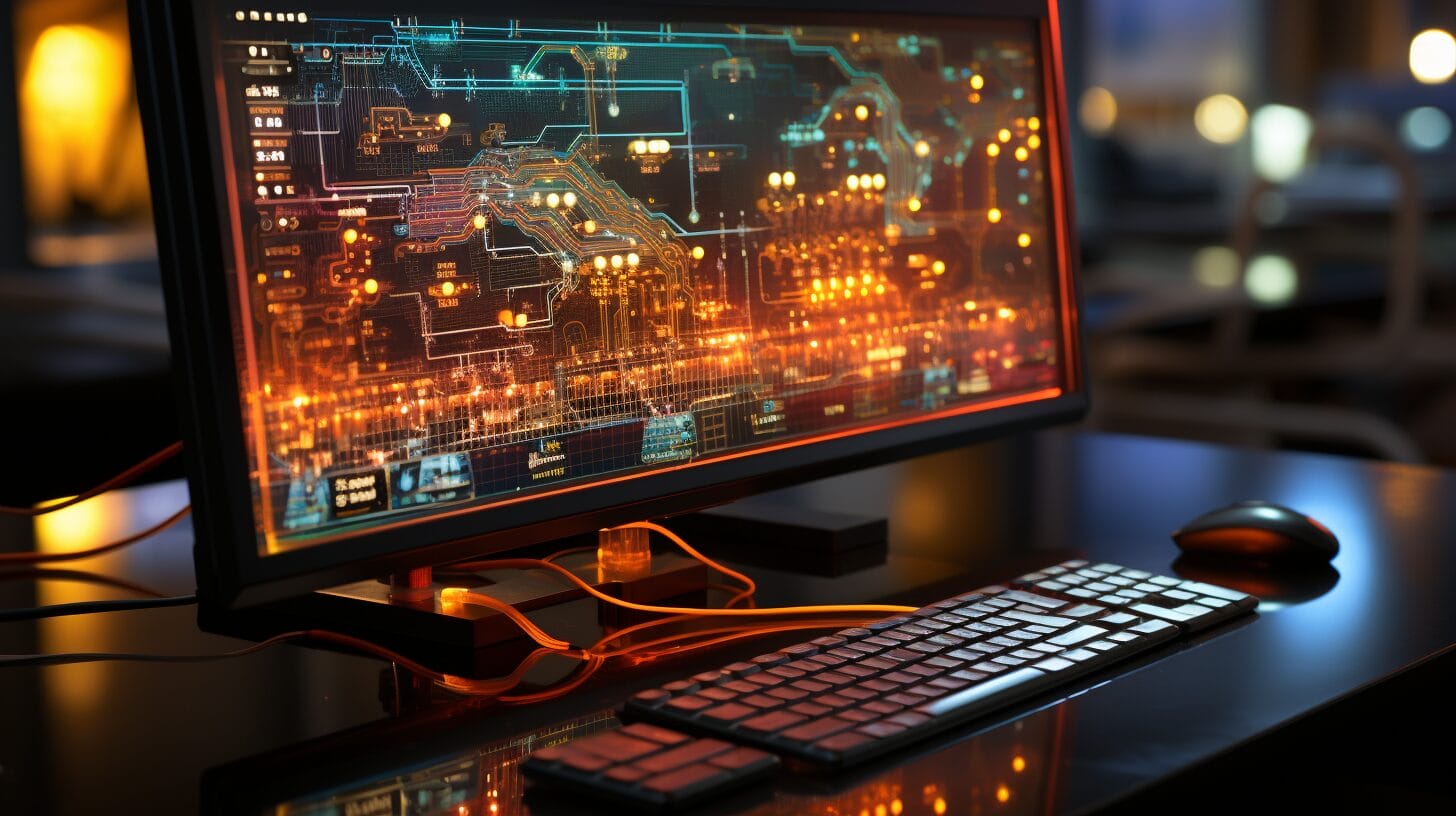
Understanding how to declare and assign values to variables in JavaScript is a fundamental skill for developers. It is the first step toward creating dynamic user interactions.
A variable in JavaScript is a storage container for data values. They can be declared using var
, let
, or const
, each with unique characteristics:
var
provides function scope.let
introduces block scope for safer code.const
ensures the immutability of a variable’s value.
Here’s how to declare and assign values:
“`javascript // Declaring a variable let freedom;
// Assigning a value to a variable freedom = ‘expression’;
let message = ‘Hello, world!’; console.log(message); // Outputs: Hello, world!
Using console.log()
, we can observe our variables in action, marking an essential step towards creating interactive web experiences.
Display Variables in HTML Content Using JavaScript
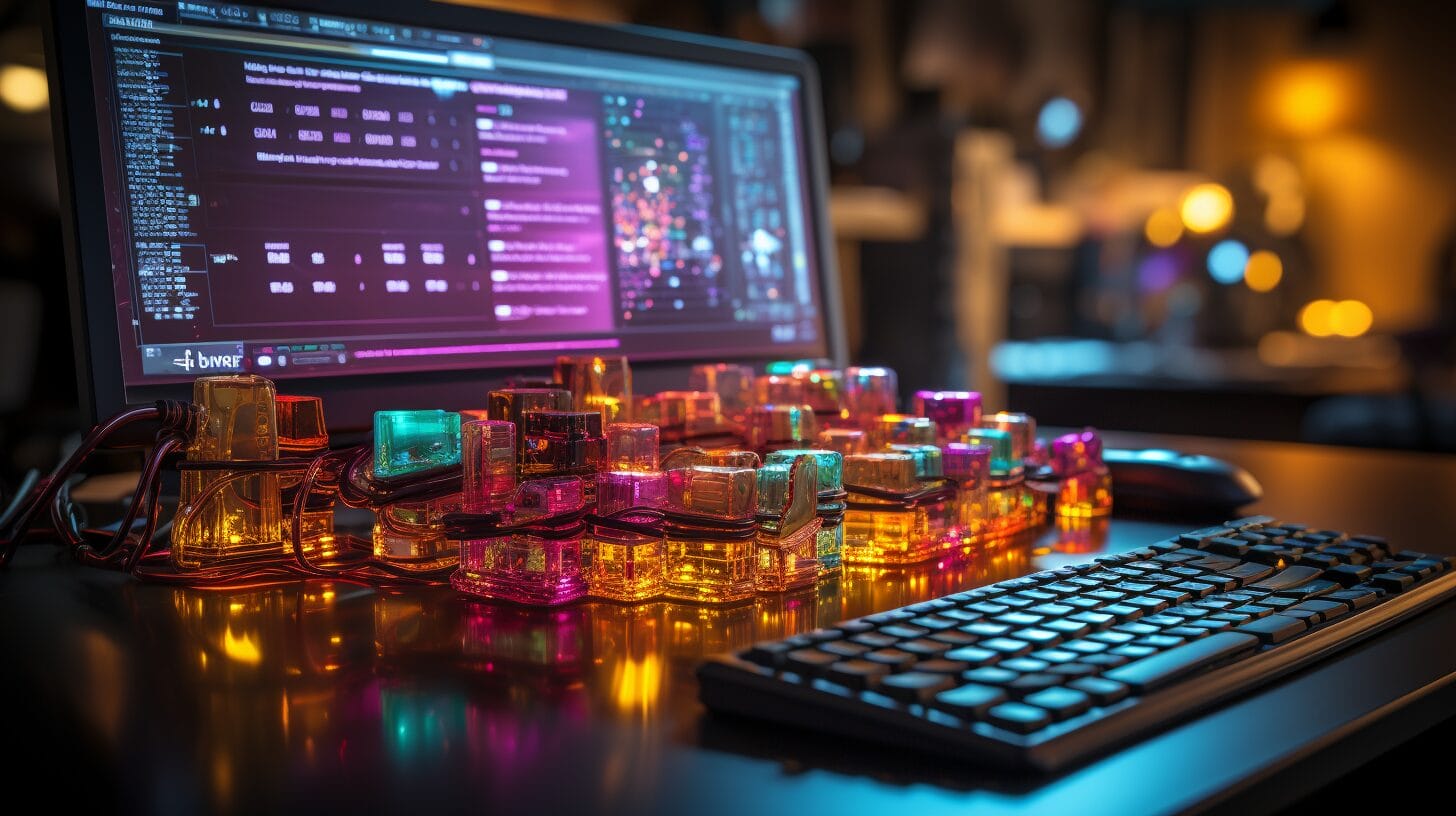
JavaScript can infuse static HTML with dynamic content, transforming standard pages into personalized experiences.
Displaying variables on web pages involves targeting the appropriate elements and accurately assigning values. The console.log
function is a crucial tool in the debugging process, allowing developers to verify the state of their variables at any stage in the code.
It is also important to ensure that content updates are accessible, taking into consideration how changes are perceived by screen readers and other assistive technologies.
Displaying variables on web pages involves targeting the appropriate elements and accurately assigning values. The console.log
function is a crucial tool in the debugging process, allowing developers to verify the state of their variables at any stage in the code.
It is also important to ensure that content updates are accessible, taking into consideration how changes are perceived by screen readers and other assistive technologies.
Mastering the Console.Log Function to Print Variables in JavaScript
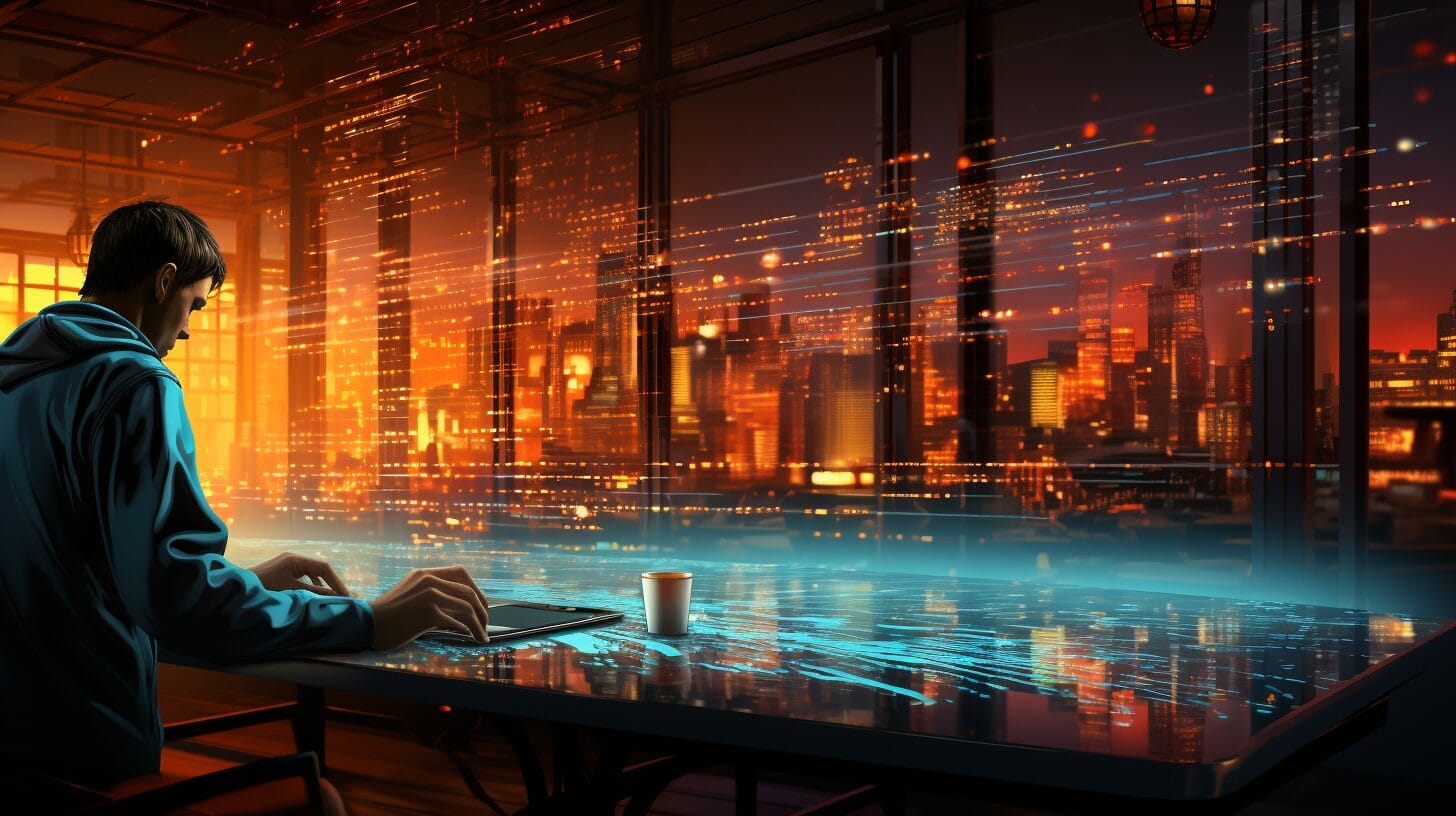
The console.log
function is indispensable for developers to effectively display variable values and facilitate the debugging process. It is a tool that opens the door to greater clarity and control over code.
To maximize the use of console.log
, follow these guidelines:
- For basic usage, pass the variable directly to
console.log(variable)
. - To print multiple variables, separate them with commas, like
console.log(var1, var2)
. - For advanced techniques, combine strings and variables for more descriptive logs, or use
%s
or%d
as placeholders for strings or numbers, respectively.
Be aware of common pitfalls, such as printing undefined variables, and consider the console’s limitations with asynchronous code. print variables in javascript With asynchronous JavaScript code, the timing of operations matters a lot.
Since console.log() prints at the exact moment it’s called, variables might not have the expected values yet in asynchronous logic. We should account for latency with APIs, promises, callbacks, etc.
Logging values too early could print undefined or stale data. Carefully considering the time and sequence of our console output avoids false assumptions.
Harnessing JavaScript, HTML, and CSS for Effective Web Development
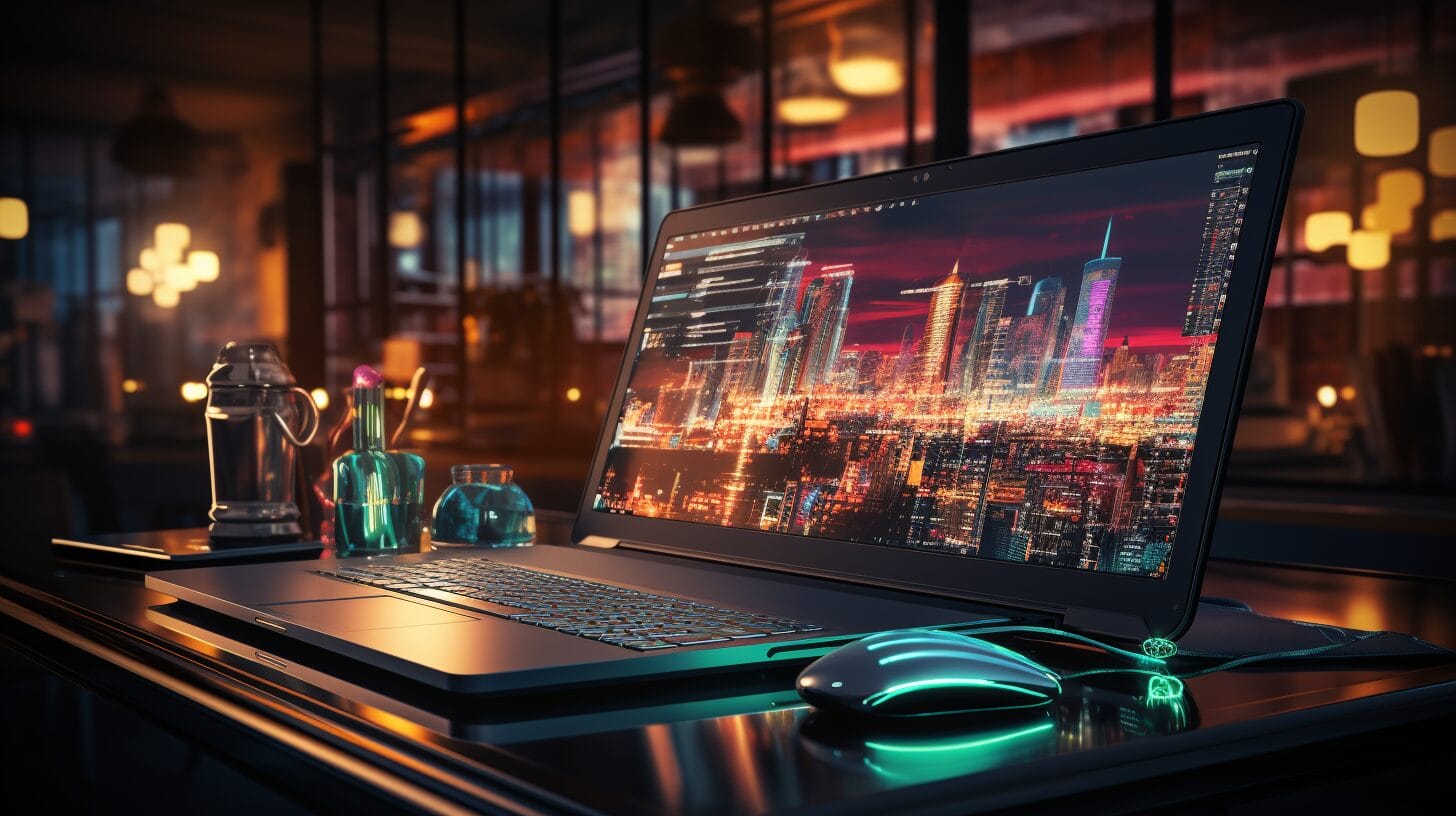
In the triad of web development, JavaScript adds the dynamic element to the structure provided by HTML and the style of CSS. This combination allows developers to craft rich, responsive user experiences.
With JavaScript, we can animate HTML elements, creating a seamless dialogue between the user and the interface. Using console.log
, we can troubleshoot to ensure scripts perform flawlessly.
CSS adds the final aesthetic touch, influencing not just the functionality but also the visual appeal of the web experience. print variables in javascript by using CSS to style and layout pages that display variable values printed with console.log(), we can create interfaces both practical and beautiful.
As we bring visual polish to utils like charts, calculators and interactive widgets powered behind the scenes by JavaScript, we transform raw data into engaging end-user experiences.
Can JavaScript Variables Be Used to Create CSS Graphs and Charts?
Yes, JavaScript variables can be used to create CSS graphs and charts. By following CSS graph and chart tutorials, you can learn how to manipulate and update variables in JavaScript to dynamically generate and visualize data in the form of graphs and charts using CSS.
Conclusion
Our exploration of the console has enhanced our JavaScript capabilities. We have tackled variables, traced bugs, and benchmarked performance.
Now, armed with the knowledge to seamlessly print variables in javascript to the console, we are prepared to weave JavaScript, HTML, and CSS into web development masterpieces.
Let’s embrace this expertise as we continue creating code that is robust and insightful. Happy printing variables and debugging, artisans of the web!
Frequently Asked Questions
How can I define a javascript variable?
To define a javascript variable, you use the ‘var’, ‘let’, or ‘const’ keywords before the variable name. printvariablesinjavascript For example: ‘var myVariable;’, ‘let myVariable;’ or ‘const myVariable = 10;’. Once you have defined a variable, you can print its value to the browser console using console.log():
let name = "John";
console.log(name);
This will print “John” to the console.
You can also print multiple variables in one statement:
“`js
let age = 30;
console.log(name, age);
“`
This will print both the name and age variables to the console.
So console.log() is very useful for printing variable values that you have defined in JavaScript using var, let or const. It helps debug code and see the values stored in your variables.
How can I display a javascript variable value to the console?
To display a JavaScript variable value to the console, you can use the console.log function. printvariablesinjavascript It is a method in JavaScript used to print output or to print a variable. For example: ‘console.log(myVariable);’. To print multiple variables, you can pass them into console.log() separated by commas:
let name = "John";
let age = 30;
console.log(name, age);
This will print both the name and age variables to the console in one statement.
You can also concatenate strings and variables together in the console.log() using +:
js console.log("Name: " + name + ", Age: " + age);
This prints a string containing the variable values.
So in summary, console.log() is very versatile for printing variables and output in JavaScript. It’s an essential tool for debugging code.
What is the way to print a Javascript variable in a browser?
To print a Javascript variable in a browser, you can use the ‘console.log()’ method. printvariablesinjavascript In addition, JavaScript using HTML can interact and manipulate the HTML pages. Hence, you can also use ‘document.write()’ to print the output directly to HTML. For example:
let name = "John";
console.log(name);
document.write(name);
This will print the variable name “John” both to the browser console using console.log() and directly to the HTML page using document.write().
Some key differences are that console.log() prints to the console, while document.write() prints to the HTML page. Also, document.write() can cause the entire page to be replaced, while console.log() just outputs to the console which is useful for debugging.
So in summary, use console.log() to print variables in JavaScript to the browser console for debugging/testing and document.write() to print directly to HTML page.
How can I initialize an array in Javascript?
To initialize arrays in JavaScript, you can use the ‘var’ keyword followed by the variable name, equal sign and square brackets. For example: ‘var myArray = [];’. To print variables in javascript you can print out the values in a JavaScript array using console.log(). For example:
var myArray = [1, 2, 3];
console.log(myArray);
This will print out the entire array [1, 2, 3] to the console.
You can also print out individual elements of the array using their index. For example:
console.log(myArray[0]);
This will print just the first element of the array (1) to the console.
So console.log() is very handy for printing JavaScript arrays and inspecting their values. It’s useful for debugging and testing code that involves arrays.
How can I display Javascript code on my HTML page?
To display Javascript code on your HTML page, use the ‘script’ tag. The JavaScript code can be placed between the ‘script’ tags. For example: ”. To print variables in javascript you can use console.log() to print variables in JavaScript. This will output the variables to the browser console. For example:
let name = "John";
console.log(name);
This will print the value of the name variable (“John”) to the console. You can print multiple variables like:
let age = 30;
console.log(name, age);
This prints both the name and age variables. console.log() is very useful for debugging JavaScript code.