Node Class in Python: Master the Fundamentals of Linked Lists Creation in 2024
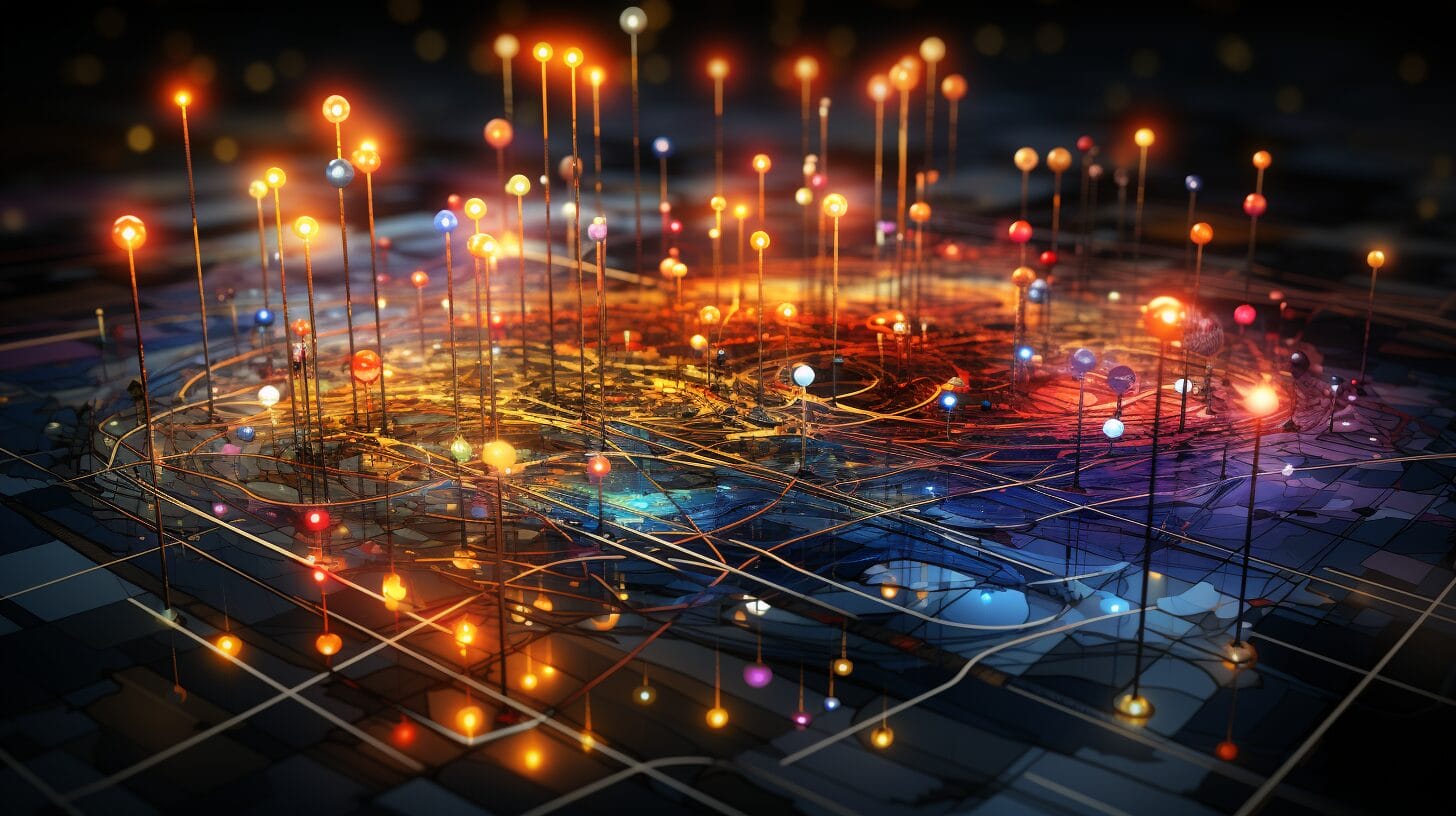
We are embarking on a voyage across the digital oceans, navigating through the well-known process of building data structures, specifically focusing on Python’s Node class.
While the array might be the trusty compass for many programmers, the allure of linked lists for their prowess in navigating dynamic data can’t be ignored.
Our mission is to demystify the art of crafting these linked lists by constructing a sturdy Node class. As we piece together the elements of this fundamental structure, we’ll uncover the secrets that make linked lists a go-to choice for modern coding challenges.
By the end of this discussion, you’ll know to not only understand the intricacies of linked lists but also to leverage their potential to optimize your programming projects.
Key Takeaways
- The Node class is essential for creating linked lists in Python.
- Linked lists allow for efficient insertion and deletion without contiguous memory space.
- Singly linked lists have two attributes: data and a reference to the next node.
- The Node class can be customized for different data structures and is the foundation of an efficient and adaptable graph.
Understanding Node Class in Python
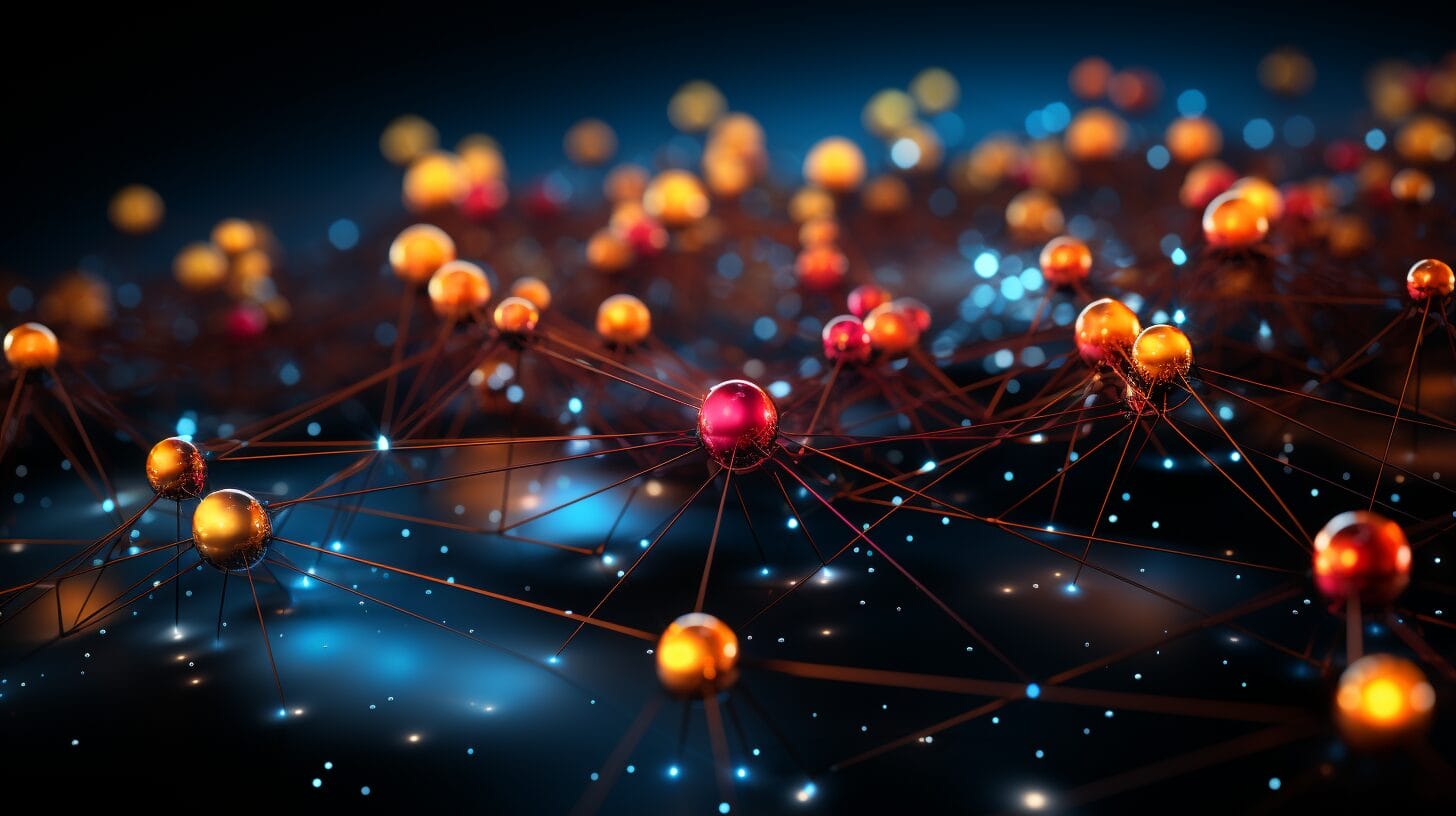
In Python, a Node class acts as the fundamental building block for creating linked lists, distinguishing them from other data structures through its unique ability to store data and a reference to the next node. This dual capacity grants us the freedom to build dynamic and flexible data structures that aren’t confined by the strictures of conventional arrays.
The node class creates standalone objects with data attributes to store values and “next” pointer attributes to link nodes sequentially. Chaining node pointers together forms a linked list that allows efficient insertion and deletion without requiring contiguous memory. We can customize node classes to fit specific data needs whether a simple to-do list or complex graph.
Anatomy of a Singly Linked List Node in Python
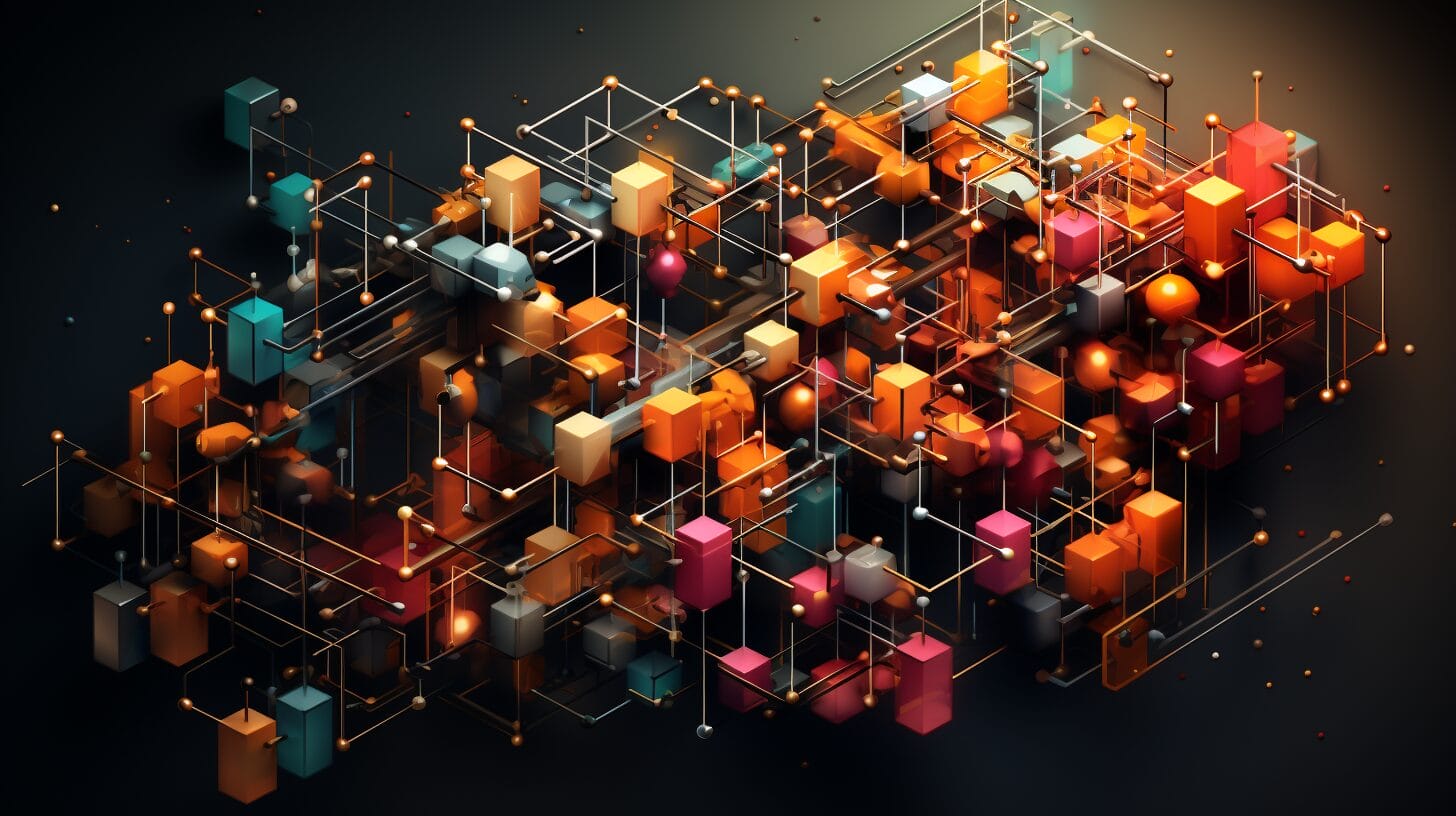
A singly linked list is a sequence of nodes where each node holds data and a reference to the next node in the list. This structure allows for dynamic memory usage and efficient insertions or deletions without the need to shift elements as in an array.
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
In this class node, data can be any data type – a number, a string, an object, or even another linked list, giving you the freedom to store complex information. The next attribute points to the next node in the list, which means you have the power to chain nodes together however you see fit.
Understanding the anatomy of a singly linked list node in Python empowers us to create versatile and dynamic data structures tailored to our specific needs, ensuring we’re not confined by the limitations of static arrays.
Implementation of Node Class: From Basics to Advanced
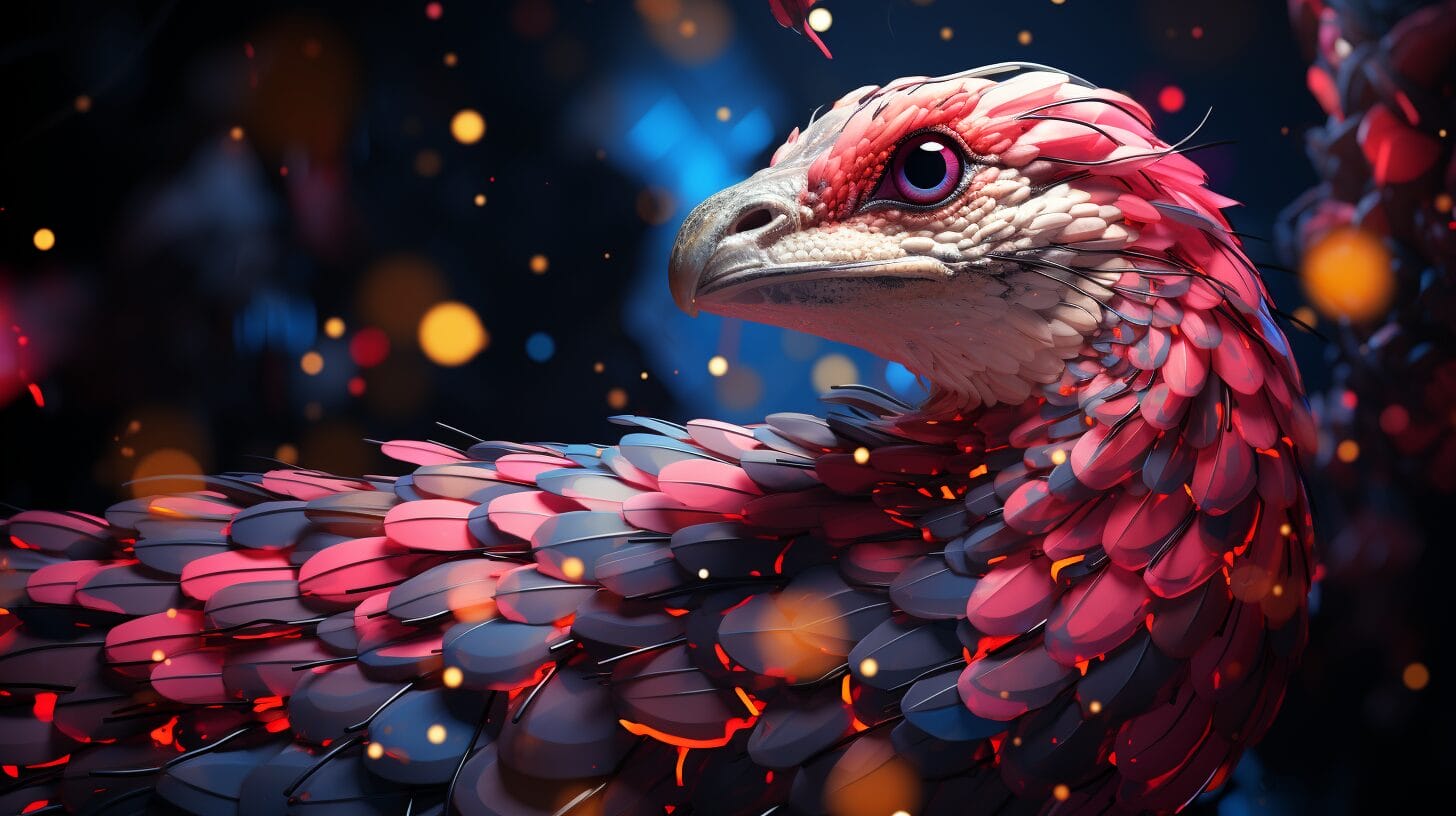
The implementation of the Node class, progressing from basic setup to mastering advanced operations such as insertion, deletion, and searching within a singly linked list, is a journey into the versatility of Python’s linked list data structure.
Here are the key points about linked lists in bullets:
- Create a node by defining a node class with data and next attributes
- Once node class set up, can add nodes to linked list flexibly
- Deletion is easy – just change the pointer to remove a node
- Searching has simplicity of linear search through nodes
- Start by creating a new node with data and link to next node
- Then build out linked list by adding nodes pointing to each other
Mastering these operations in Python is about understanding the freedom that a dynamic data structure can offer, both in terms of memory usage and operations efficiency.
Graph Nodes: Advanced Node Class Implementation in Python
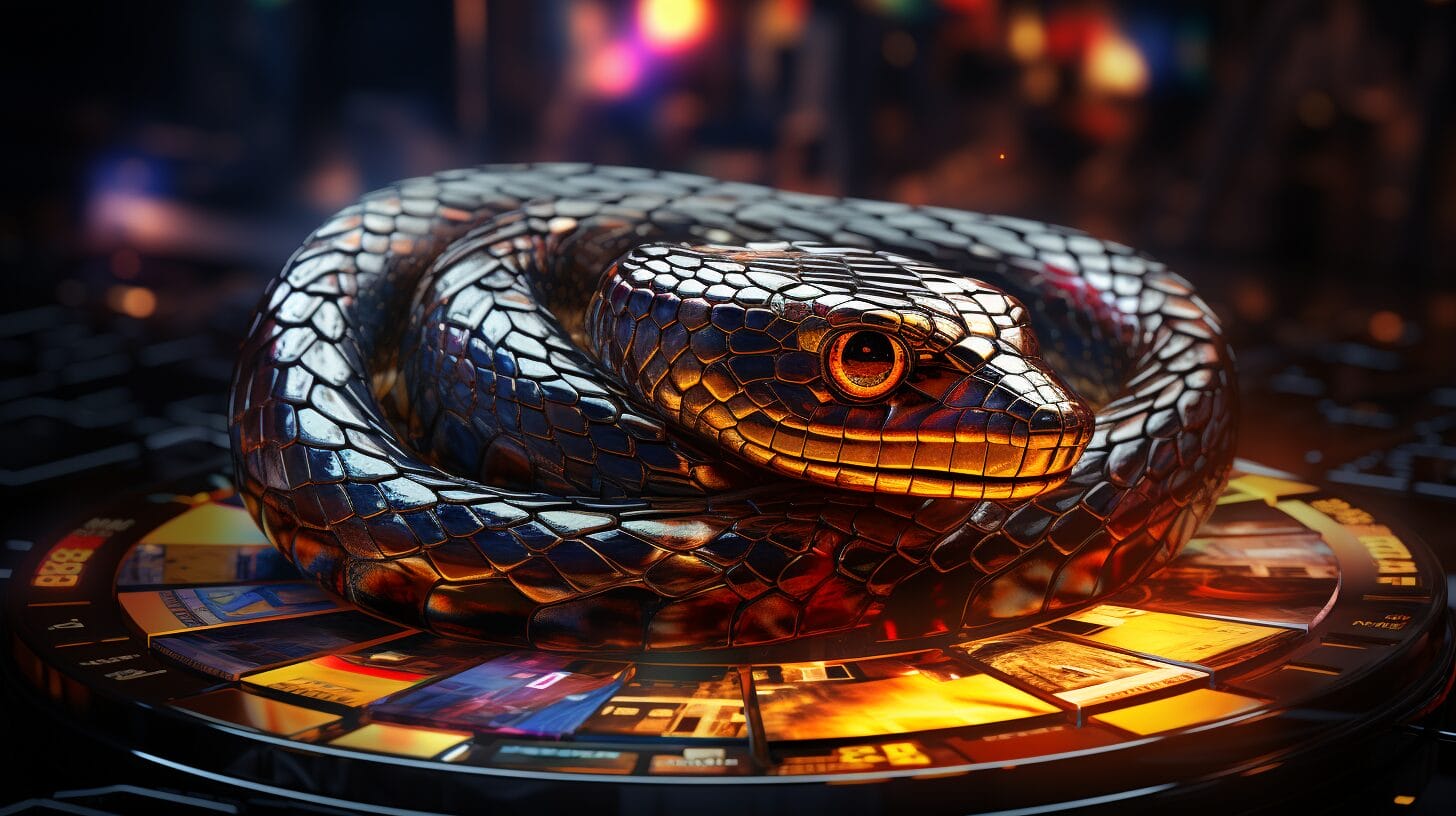
Here are the key points about the advanced graph node class implementation in bullet points:
- Graph nodes can have multiple connections, mirroring real-world networks
- We implement an advanced node class in Python to manage connections
- Graphs are non-linear structures representing complex relationship
- Our node class handles dynamic connections efficiently
- Supports efficient insertion and deletion of connections
- Graph traversal methods like DFS and BFS return connected nodes
- Useful for analyzing or searching graphs with intricate connections
In our quest for freedom within Python data structures, we’re not just creating isolated points; we’re building a web of interconnected nodes. Our advanced node class is the foundation of an efficient and adaptable graph.
How Does Understanding Linked Lists Creation in Python Fit into Learning Object Oriented Languages?
Understanding linked lists creation in Python is essential for mastering object-oriented languages like Java, C++, and C#. Python’s simplicity and syntax make it a great language to start with when learning about the ultimate object oriented language list. grasping linked lists in Python lays a strong foundation for understanding advanced data structures and OOP concepts.
Node Class in Python: Common Errors and Debugging
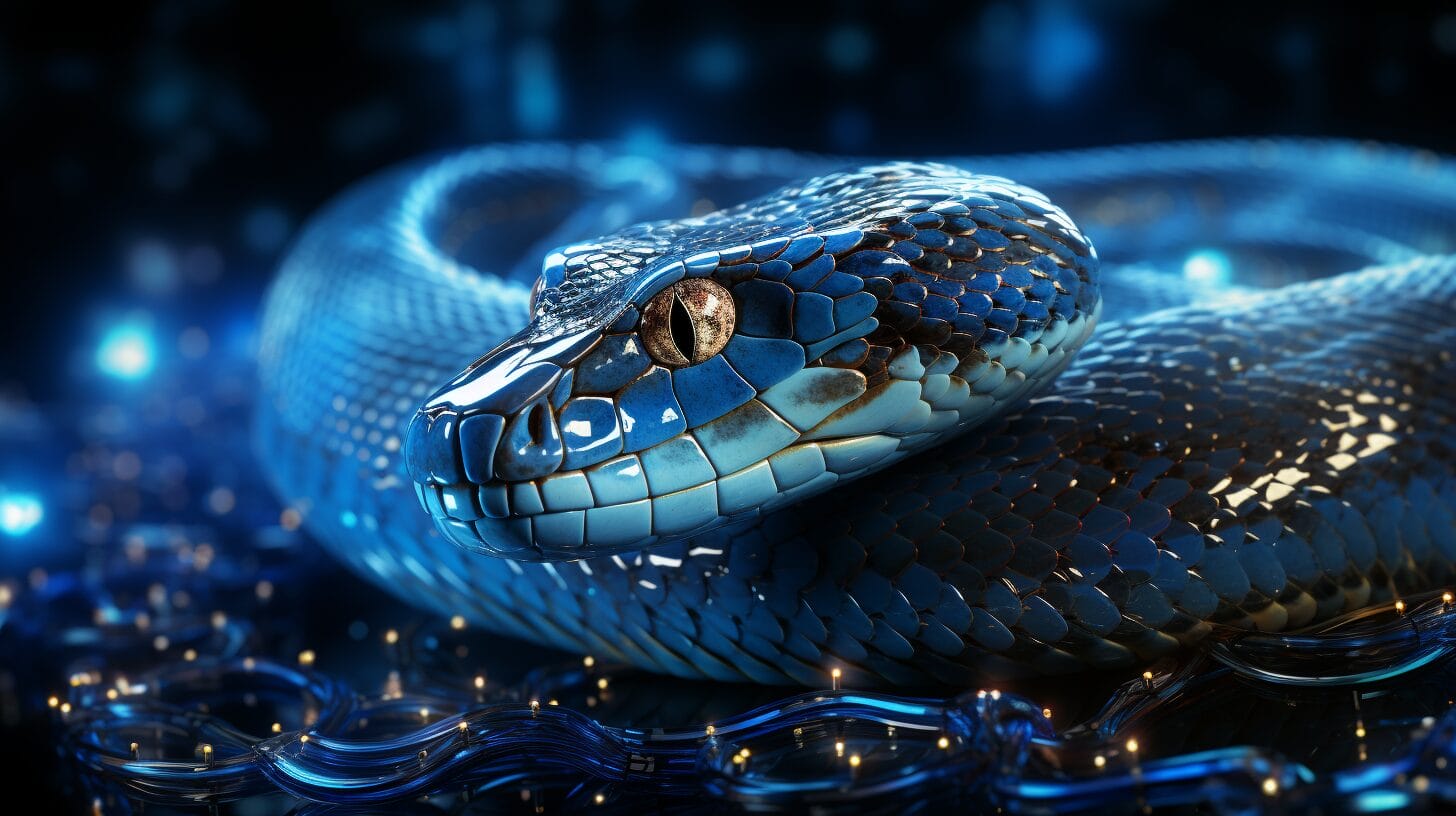
Crafting a node class in Python offers the liberty to structure our data innovatively. A frequent misstep is neglecting the initial setting of the next pointer to None in the constructor of a node class. We’ve also seen instances where the node’s data isn’t properly encapsulated, which can cause unexpected data manipulation and lead to hours of debugging.
When debugging, it’s helpful to print out the linked list’s elements and use Python’s built-in debugging tools.
Conclusion
We’ve navigated the complexities of linked lists in Python, skillfully crafting our Node class from the ground up. Our coding journey has not only bolstered our understanding but also primed us for tackling more complex challenges. With common pitfalls behind us, we’re now equipped to weave nodes into intricate data tapestries.
Let’s celebrate our triumph and keep coding with confidence!
Frequently Asked Questions
What is a Node class in Python?
A Node class in Python is a fundamental building block for creating linked lists. It represents an individual element in the linked list, containing data and a reference to the next node in the list.
How do you implement a Node class in Python?
To implement a Node class in Python, you can define a class with attributes to store data and a reference to the next node. For example: “`python class Node: def __init__(self, data): self.data = data self. next = None “` This code snippet creates a basic Node class with data and a next reference initialized to None.
What is the significance of the Node class in data structure?
The Node class is significant in data structures, particularly in the context of linked lists. It allows for the creation of a linked list data structure, where each node is connected to the next, forming a sequence.
How can I return a list of nodes from a linked list in Python?
You can return a list of nodes from a linked list in Python by traversing the entire list and storing each node in a Python list or another data structure. This allows you to obtain a collection of all the nodes in the linked list.
What is the role of the head node in a linked list created using the Node class in Python?
The head node serves as the initial starting point of a linked list created using the Node class in Python. It is the first node in the list and acts as the entry point for traversing the entire list.